隐式启动Activity时,Android系统在应用程序运行时解析Intent,并根据一定的规则对Intent和Activity进行匹配,使Intent上的动作、数据与Activity完全吻合。而匹配的Activity可以是应用程序本身的,也可以是Android系统内置的,还可以是第三方应用程序提供的。因此,这种方式更强调了Android应用程序中组件的可复用性。
由此可以看出,隐式启动不需要指明需要启动哪一个Activity,而由Android系统来决定,有利于使用第三方组件。
在默认情况下,Android系统会调用内置的Web浏览器,代码如代码清单6-5所示。
代码清单6-5 隐式启动默认情况调用内置Web浏览器
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse("//www.google.com"));
startActivity(intent);
在上述代码中,Intent的动作是Intent.ACTION_VIEW,是根据Uri的数据类型来匹配动作;数据部分的Uri是Web地址,使用Uri.parse(urlString)方法,可以简单地把一个字符串解释成Uri对象。
Intent的语法如代码清单6-6所示。
代码清单6-6 Intent语法
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(urlString));
Intent构造函数的第1个参数是Intent需要执行的动作;第2个参数是Uri,表示需要传递的数据。
Android系统支持的常见动作字符串常量表,如表6-3所示。
表6-3 Android系统支持的常见动作字符串常量表
动 作 |
说 明 |
ACTION_ANSWER |
打开接听电话的Activity,默认为Android内置的拨号盘界面 |
ACTION_CALL |
打开拨号盘界面并拨打电话,使用Uri中的数字部分作为电话号码 |
ACTION_DELETE |
打开一个Activity,对所提供的数据进行删除操作 |
ACTION_DIAL |
打开内置拨号盘界面,显示Uri中提供的电话号码 |
ACTION_EDIT |
打开一个Activity,对所提供的数据进行编辑操作 |
ACTION_INSERT |
打开一个Activity,在提供数据的当前位置插入新项 |
ACTION_PICK |
启动一个子Activity,从提供的数据列表中选取一项 |
ACTION_SEARCH |
启动一个Activity,执行搜索动作 |
ACTION_SENDTO |
启动一个Activity,向数据提供的联系人发送信息 |
ACTION_SEND |
启动一个可以发送数据的Activity |
ACTION_VIEW |
常用的动作,对以Uri方式传送的数据,根据Uri协议部分以佳方式启动相应的Activity进行处理。对于http:address将打开浏览器查看;对于tel:address将打开拨号呼叫指定的电话号码 |
ACTION_WEB_SEARCH |
打开一个Activity,对提供的数据进行Web搜索 |
以下将通过一个WebViewIntentDemo示例来了解如何隐式启动Activity。
如图6-3所示,当用户在文本框中输入要访问的网址后,通过单击“Go”按钮,程序根据用户输入的网址生成一个Intent,并以隐式启动的方式调用Android内置的Web浏览器,并打开指定的Web页面。本例输入的网址是google主页的主站地址,地址是://www.google.com。
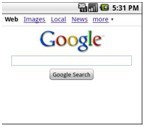
图6-3 隐式启动Activity
其中,main.xml代码如代码清单6-7所示。
代码清单6-7 main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="//schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<EditText
android:id="@+id/url_field"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1.0"
android:lines="1"
android:inputType="textUri"
android:imeOptions="actionGo" />
<Button
android:id="@+id/go_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/go_button" />
</LinearLayout>
其中,strings.xml代码如代码清单6-8所示。
代码清单6-8 strings.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name"> WebViewIntentDemo </string>
<string name="go_button">Go</string>
</resources>
WebViewIntentDemo.java代码如代码清单6-9所示。
代码清单6-9 WebViewIntentDemo.java
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.KeyEvent;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.View.OnKeyListener;
import android.widget.Button;
import android.widget.EditText;
public class WebViewIntentDemo extends Activity {
private EditText urlText;
private Button goButton;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// Get a handle to all user interface elements
urlText = (EditText) findViewById(R.id.url_field);
goButton = (Button) findViewById(R.id.go_button);
// Setup event handlers
goButton.setOnClickListener(new OnClickListener() {
public void onClick(View view) {
openBrowser();
}
});
urlText.setOnKeyListener(new OnKeyListener() {
public boolean onKey(View view, int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_ENTER) {
openBrowser();
return true;
}
return false;
}
});
}
}
在上述代码中第26行对按钮“Go”添加监听,当触摸单击或通过手机按键单击该按钮时,触发openBrowser()方法。其中,openBrowser()方法代码如代码清单6-10所示。
代码清单6-10 openBrowser()方法
/** Open a browser on the URL specified in the text box */
private void openBrowser() {
Uri uri = Uri.parse(urlText.getText().toString());
Intent intent = new Intent(Intent.ACTION_VIEW, uri);
startActivity(intent);
}